Process
This project was completed throughout my sophomore spring semester at Carnegie Mellon University in 67-272 Application Design and Development. With the guidance of our professor, each student completed their own copy of the project while meeting rigorous requirements discussed below.
01
Use Cases
Use cases guided development from start to finish.
Based on a short three-page description, I began the project by identifying a set of use cases. These cases were categorized as A, B, or C-level in order of importance and matched with a short list of actors who would be interacting with the app. This step was crucial in guiding my work to its completion since it allowed me to plan ahead and develop in a time-efficient manner without forgetting important functionality.
02
Data Design
The database was built after defining the data and making an entity-relationship diagram.
Before setting up the database, I took important precautions to ensure it would be robust long-term and fit the needs of the application. I began with an entity-relationship diagram to denote the relational structure of the database as well as record certain limitations in the cardinality of each relation. This diagram was then normalized into third normal form to prevent data anomalies and converted to a relational schema containing notes on implementation. Finally, I compiled a data dictionary that further detailed data types and better described the purpose of each attribute. These three documents affirmed that I made the database correctly upon the first implementation.
03
MVC Framework
The model, view, and controller were each developed at separate stages of the process.
Using the framework provided with Ruby on Rails, my application followed the MVC standard of software development. Once the database was created, I began writing the code for the models, which interact directly with the database and aid in filtering and verifying data integrity. After unit testing the models, work continued in the controller. This staged process helped me know that any new errors were isolated to the controller, helping with debugging. The controller would pass data from the model to the appropriate view page, developed with Rails's built-in template engine.
04
Wireframe Prototyping
Great design starts with rough sketches.
The application was built using Google's Materialize CSS framework that contributed a pre-defined set of styles and classes. But aspects such as page layout required additional design, which is where I incorporated wireframe prototyping. By quickly sketching several ideas, I identified the best features to include in my final design and didn't waste time manually programming a poor interface. I moved from low-fidelity sketches to Adobe XD designs that included annotations to better explain my design choices. These prototypes were especially beneficial for the user dashboards, which contain information custom to the employee's role at the company.
05
Design
Designed to increase speed and reduce cognitive load.
Designing for the user often means thinking about the small details that refine one's experience. To be successful, I needed the application to be quick and stress-free. If an operation is impossible - say, editing your pay grade as an employee - the option is hidden from view rather than disabled to prevent frustration. Default values were included in as many forms as possible, while other data persisted between pages to remove repeated data entry. For example, when generating payroll from a store's info page, the application provides a default date range for the past two weeks and calulates only the employees at that store. After generation, these filters are included on the payroll in case the manager forgets what inputs were used. Alert messages are used frequently throughout the site to confirm actions are completed successfully.
Most importantly, each user's dashboard contained information relevant to them. Company owners could easily reassign employees from over-staffed stores, generate payroll for a specific store, and see if any employees are freqeuntly missing shifts. Manager dashboards provided a quick link to schedule shifts to employees and view today's schedule so they know who to expect in the store. Employees always had quick access to their upcoming schedule and the amount of their most recent paycheck. These dashboards used basic shift data, merged it with several other tables, and then filtered it to reveal valuable insight for each role.
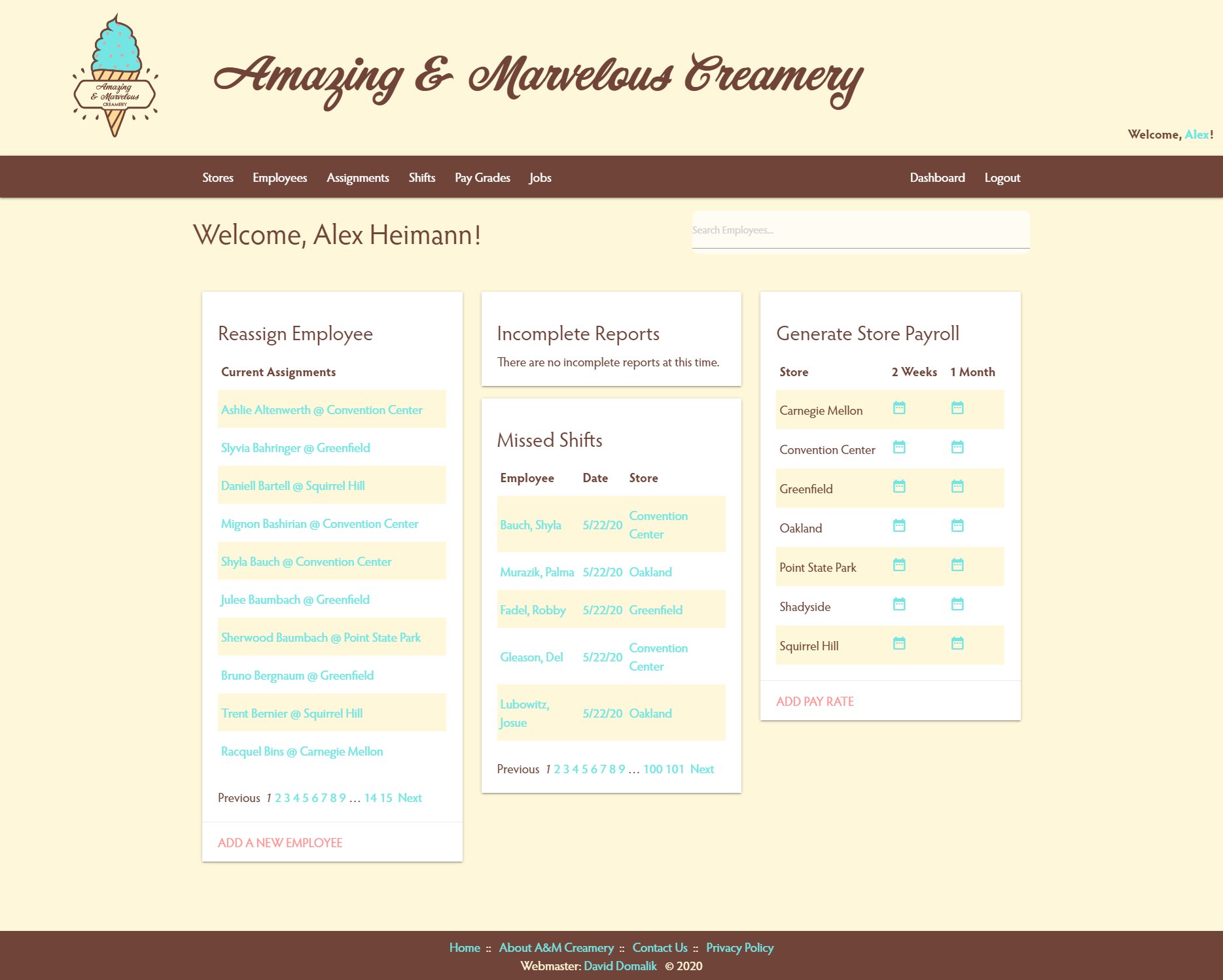
Admin Dashboard
06
Gem Integration
Increased functionality and improved code style with Ruby gems.
Ruby on Rails has hundreds of plug-ins known as "gems" that can be easily imported for use in your program. In my application, gems like simple_form and validates_timeliness aided in form construction and date/time input validation. CanCanCan managed authorization and authentication in a secure fashion. will_paginate increased page load times and reduced content overload by adding pagination to the site. Then a handful of testing gems helped generate fake testing data and controlled both unit and automated testing. Finally, simplecov ensured the website had 100% test coverage.
07
100% Test Coverage
No part of the application is left untested.
A strict requirement of the project was to meet 100% test coverage, meaning every line of code is touched by my unit tests. I wrote tests for both the models and controllers. Cucumber tests were provided for our views, allowing me to experiment with test-driven development. With 100% coverage, I can be confident my program is fully-functional with minimal bugs.